Word Processing Control
Overview
The "Word processing" control is an evolved control allowing the end user to create and edit documents in docx format in theWINDEV application. The benefits are time saving, consistent interface, no other software to install/drive/manage.
This control allows you to:
- · Open, create, modify, save "docx" files,
- · Convert docx into PDF, into HTML to send emails,
- · Manage the images and tables,
- · Save the documents in HFSQL databases,
- · Use a spelling checker,
- · Perform prints, ...
Several keyboard shortcuts are also available:
Shortcut
|
Effect
|
Shortcut
|
Effect
|
CTRL A
|
Selects the entire text.
|
CTRL C
|
Copies the selection into the clipboard.
|
CTRL F
|
Starts the search.
|
CTRL G
|
Switches the selection to bold
|
CTRL H
|
Starts find/replace
|
CTRL I
|
Switches the selection to italic
|
CTRL S
|
Saves the document
|
CTRL U
|
Switches the selection to underlined
|
CTRL V
|
Pastes the clipboard content.
|
CTRL X
|
Cust the selection and places it in the clipboard.
|
CTRL Y
|
Redo the last action.
|
CTRL Z
|
Undo the last action.
|
CTRL mouse wheel
|
Changes the zoom.
|
CTRL 0
|
Zoom the document at 100%
|
CTRL home
|
Moves up to the start of document.
|
CTRL End
|
Displays the end of document.
|
Page Up
|
Displays the previous page.
|
Page down
|
Displays the next page.
|
To create a Word Processing control:
1. On the "Creation" pane, in the "Graphic controls" group, click "Word processing".
2. Click the position where the control will be created in the window.
Note: The dimensions of the created control are optimized in order to occupy the available space at the specified position. If the control size does not suit your, press [CTRL Z]: the default size of control will be restored.
To display the control characteristics, select "Description" from the popup menu of control.
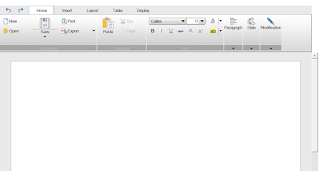
Handling the Word Processing control by programming
To open an existing docx document by programming in a Word Processing control, you can:
· assign the Word Processing control with the docx file. For example:
// Selects a .docx file in the current directory
sFileName is string
sFileName = fSelect("", "presentation.docx", ...
"Selecting DocX files", ...
"Docx file (*.docx)" + TAB + "*.docx", "*.docx", fselOpen)
IF sFileName ~="" THEN
// No selected file
RETURN
END
// Load the docx file
WP_MyDocument = sFileName
sFileName is string
sFileName = fSelect("", "presentation.docx", ...
"Selecting DocX files", ...
"Docx file (*.docx)" + TAB + "*.docx", "*.docx", fselOpen)
IF sFileName ~="" THEN
// No selected file
RETURN
END
// Load the docx file
WP_MyDocument = sFileName
· use DocOpen. For example:
WP_MyDocument = DocOpen("C:\Users\test\Documents\file.docx")
IF ErrorOccurred() THEN
Error(ErrorInfo())
RETURN
IF ErrorOccurred() THEN
Error(ErrorInfo())
RETURN
· Caution: only the files in docx format can be handled by the Word Processing control.
Tip: To display an empty document in a Word Processing control, all you have to do is assign an empty string to the Word Processing control.
Example:
Example:
// Create a blank document
WP_MyDocument = ""
WP_MyDocument = ""
To save a docx document, all you have to do is use DocSave.
Example:
// Choose the directory and the backup name
sFileName is string = fSelect(CompleteDir(fExeDir()),"presentation.docx", ...
"Selecting DocX files","Docx file (*.docx)" + TAB + "*.docx" , "*.docx", fselCreate)
// Save the file
DocSave(WP_NoName1, sFileName)
IF ErrorOccurred THEN
Error(StringBuild("The %1 file was not saved.", sFileName), ErrorInfo())
RETURN
END
sFileName is string = fSelect(CompleteDir(fExeDir()),"presentation.docx", ...
"Selecting DocX files","Docx file (*.docx)" + TAB + "*.docx" , "*.docx", fselCreate)
// Save the file
DocSave(WP_NoName1, sFileName)
IF ErrorOccurred THEN
Error(StringBuild("The %1 file was not saved.", sFileName), ErrorInfo())
RETURN
END
Notes:
· The content of the Word Processing control can also be:
· printed (iPrintDoc).
· saved in PDF format (DocToPDF).
· DocToText is used to retrieve the document text.
· DocToImage is used to convert a document page into image.
To perform a search in a Word Processing document, all you have to do is use DocSeek.
Example:
// Seeks "BEAUTIFUL" in the text
// Selects the first one found to change its color
arrFragments is array of DocFragments = DocSeek(WP_NoName1, "BEAUTIFUL")
IF arrFragments..Occurrence >= 1 THEN
// Position the cursor at the beginning of the first word found
WP_NoName1..Cursor = arrFragments[1]..StartPosition
// Calculate the selection length
WP_NoName1..SelectionLength = arrFragments[1]..EndPosition - arrFragments[1]..StartPosition+1
// Modify the text color
arrFragments[1]..Formatting..TextColor = PastelRed
BREAK
END
// Selects the first one found to change its color
arrFragments is array of DocFragments = DocSeek(WP_NoName1, "BEAUTIFUL")
IF arrFragments..Occurrence >= 1 THEN
// Position the cursor at the beginning of the first word found
WP_NoName1..Cursor = arrFragments[1]..StartPosition
// Calculate the selection length
WP_NoName1..SelectionLength = arrFragments[1]..EndPosition - arrFragments[1]..StartPosition+1
// Modify the text color
arrFragments[1]..Formatting..TextColor = PastelRed
BREAK
END
In this code, the DocFragment variable corresponds to the text section containing the sought word, as well as its position in the entire text. Then, you have the ability to handle the fragment found.
To handle the selection currently performed in a Word Processing control, you have the ability to retrieve this selection in a DocFragment variable.
The following code is used to change the color of the selected text.
Example:
Example:
// Change the selection color
// Get the current selection
MySelection is DocFragment(WP_MyDocument, WP_MyDocument..Cursor, ...
WP_MyDocument..SelectionLength)
// Change the color
MySelection..Formatting..TextColor = PastelRed
// Get the current selection
MySelection is DocFragment(WP_MyDocument, WP_MyDocument..Cursor, ...
WP_MyDocument..SelectionLength)
// Change the color
MySelection..Formatting..TextColor = PastelRed
The DocFragment variable owns a specific constructor used to easily retrieve the selection.
Several methods can be used to add text into an existing document:
· Inserting a fragment at the requested position. Example:
// Inserts a text at the end of document
frag2 is DocFragment(WP_MyDoc, -1 , 0)
frag..Text += "End text"
frag2 is DocFragment(WP_MyDoc, -1 , 0)
frag..Text += "End text"
· Inserting a text from a given position with DocInsert.
DocInsert(WP_Document, WP_Document..Cursor, "My inserted text")
· Adding a text at the end of document with DocAdd.
To add text into an empty document, you can:
· use the direct assignment of control:
<Word Processing control> = "Text to insert"
Example:
WP_MyDocument = "I am the first sentence of this document."
· add the text with DocAdd.
· insert a fragment in first position. Example:
// sInitialText contains the text to insert
frag is DocFragment(WP_MyDoc, WP_MyDoc..Cursor, 0)
frag..Text += sInitialText
frag is DocFragment(WP_MyDoc, WP_MyDoc..Cursor, 0)
frag..Text += sInitialText
· insert a text from position 1 with DocInsert.
DocInsert(WP_Document, 1, "My inserted text")
The following code is used to add a page break into a document:
// Adds a page break
DocAdd(WP_NoName, Charact(12))
DocAdd(WP_NoName, Charact(12))
Comments
Post a Comment